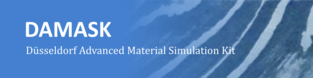 |
DAMASK with grid solvers
Revision: v2.0.3-2204-gdb1f2151
The Düsseldorf Advanced Material Simulation Kit with Grid Solvers
|
|
Go to the documentation of this file. 1 # 1 "/home/damask_user/GitLabCI_Pipeline_4301/DAMASK/src/list.f90"
4 # 1 "/home/damask_user/GitLabCI_Pipeline_4301/DAMASK/src/list.f90"
16 character(len=:),
allocatable :: val
17 integer,
dimension(:),
allocatable :: pos
55 subroutine add(this,string)
58 character(len=*),
intent(in) :: string
65 do while (
associated(temp%next))
68 temp%string%val =
io_lc(trim(string))
85 do while (
associated(item%next))
86 write(6,
'(a)')
' '//trim(item%string%val)
101 if(
associated(this%next))
deallocate(this%next)
114 if(
associated(this%next))
deallocate(this%next)
130 if (
associated(this(i)%next))
then
146 character(len=*),
intent(in) :: key
152 do while (
associated(item%next) .and. .not.
keyexists)
167 character(len=*),
intent(in) :: key
173 do while (
associated(item%next))
174 if (trim(
io_stringvalue(item%string%val,item%string%pos,1)) == trim(key)) &
187 real(pReal) function getfloat(this,key,defaultVal)
190 character(len=*),
intent(in) :: key
191 real(
preal),
intent(in),
optional :: defaultval
196 found =
present(defaultval)
200 do while (
associated(item%next))
201 if (trim(
io_stringvalue(item%string%val,item%string%pos,1)) == trim(key))
then
203 if (item%string%pos(1) < 2)
call io_error(143,ext_msg=key)
209 if (.not. found)
call io_error(140,ext_msg=key)
219 integer function getint(this,key,defaultVal)
222 character(len=*),
intent(in) :: key
223 integer,
intent(in),
optional :: defaultval
228 found =
present(defaultval)
229 if (found)
getint = defaultval
232 do while (
associated(item%next))
233 if (trim(
io_stringvalue(item%string%val,item%string%pos,1)) == trim(key))
then
235 if (item%string%pos(1) < 2)
call io_error(143,ext_msg=key)
241 if (.not. found)
call io_error(140,ext_msg=key)
252 character(len=pStringLen) function getstring(this,key,defaultVal,raw)
255 character(len=*),
intent(in) :: key
256 character(len=*),
intent(in),
optional :: defaultval
257 logical,
intent(in),
optional :: raw
261 if (
present(raw))
then
267 found =
present(defaultval)
274 do while (
associated(item%next))
275 if (trim(
io_stringvalue(item%string%val,item%string%pos,1)) == trim(key))
then
277 if (item%string%pos(1) < 2)
call io_error(143,ext_msg=key)
280 getstring = trim(item%string%val(item%string%pos(4):))
288 if (.not. found)
call io_error(140,ext_msg=key)
298 function getfloats(this,key,defaultVal,requiredSize)
302 character(len=*),
intent(in) :: key
303 real(
preal),
dimension(:),
intent(in),
optional :: defaultval
304 integer,
intent(in),
optional :: requiredsize
310 cumulative = (key(1:1) ==
'(' .and. key(len_trim(key):len_trim(key)) ==
')')
316 do while (
associated(item%next))
317 if (trim(
io_stringvalue(item%string%val,item%string%pos,1)) == trim(key))
then
320 if (item%string%pos(1) < 2)
call io_error(143,ext_msg=key)
321 do i = 2, item%string%pos(1)
328 if (.not. found)
then
329 if (
present(defaultval)) then;
getfloats = defaultval; else;
call io_error(140,ext_msg=key);
endif
331 if (
present(requiredsize))
then
343 function getints(this,key,defaultVal,requiredSize)
345 integer,
dimension(:),
allocatable ::
getints
347 character(len=*),
intent(in) :: key
348 integer,
dimension(:),
intent(in),
optional :: defaultval
349 integer,
intent(in),
optional :: requiredsize
355 cumulative = (key(1:1) ==
'(' .and. key(len_trim(key):len_trim(key)) ==
')')
361 do while (
associated(item%next))
362 if (trim(
io_stringvalue(item%string%val,item%string%pos,1)) == trim(key))
then
364 if (.not. cumulative)
getints = [
integer::]
365 if (item%string%pos(1) < 2)
call io_error(143,ext_msg=key)
366 do i = 2, item%string%pos(1)
373 if (.not. found)
then
374 if (
present(defaultval)) then;
getints = defaultval; else;
call io_error(140,ext_msg=key);
endif
376 if (
present(requiredsize))
then
391 character(len=pStringLen),
dimension(:),
allocatable ::
getstrings
393 character(len=*),
intent(in) :: key
394 character(len=*),
dimension(:),
intent(in),
optional :: defaultval
395 logical,
intent(in),
optional :: raw
397 character(len=pStringLen) :: str
403 cumulative = (key(1:1) ==
'(' .and. key(len_trim(key):len_trim(key)) ==
')')
404 if (
present(raw))
then
412 do while (
associated(item%next))
413 if (trim(
io_stringvalue(item%string%val,item%string%pos,1)) == trim(key))
then
416 if (item%string%pos(1) < 2)
call io_error(143,ext_msg=key)
418 notallocated:
if (.not.
allocated(
getstrings))
then
420 str = item%string%val(item%string%pos(4):)
425 do i=3,item%string%pos(1)
432 str = item%string%val(item%string%pos(4):)
435 do i=2,item%string%pos(1)
445 if (.not. found)
then
446 if (
present(defaultval))
then
subroutine add(this, string)
add element
pure integer function, dimension(:), allocatable, public io_stringpos(string)
locates all whitespace-separated chunks in given string and returns array containing number them and ...
subroutine show(this)
prints all elements
subroutine, public io_error(error_ID, el, ip, g, instance, ext_msg)
write error statements to standard out and terminate the Marc/spectral run with exit #9xxx
subroutine free(this)
empties list and frees associated memory
character(len=pstringlen) function, dimension(:), allocatable getstrings(this, key, defaultVal, raw)
gets array of string values of for a given key from a linked list
integer function, dimension(:), allocatable getints(this, key, defaultVal, requiredSize)
gets array of integer values of for a given key from a linked list
integer function getint(this, key, defaultVal)
gets integer value of for a given key from a linked list
integer function countkeys(this, key)
count number of key appearances
real(preal) function, dimension(:), allocatable getfloats(this, key, defaultVal, requiredSize)
gets array of float values of for a given key from a linked list
recursive subroutine finalize(this)
empties list and frees associated memory
setting precision for real and int type
logical function keyexists(this, key)
reports wether a given key (string value at first position) exists in the list
input/output functions, partly depending on chosen solver
integer, parameter preal
number with 15 significant digits, up to 1e+-307 (typically 64 bit)
logical pure function, public io_isblank(string)
identifies strings without content
subroutine finalizearray(this)
cleans entire array of linke lists
integer function, public io_intvalue(string, chunkPos, myChunk)
reads integer value at myChunk from string
pure character(len=len(string)) function, public io_lc(string)
changes characters in string to lower case
character(len=:) function, allocatable, public io_stringvalue(string, chunkPos, myChunk)
reads string value at myChunk from string
character(len=pstringlen) function getstring(this, key, defaultVal, raw)
gets string value of for a given key from a linked list
real(preal) function, public io_floatvalue(string, chunkPos, myChunk)
reads float value at myChunk from string
real(preal) function getfloat(this, key, defaultVal)
gets float value of for a given key from a linked list