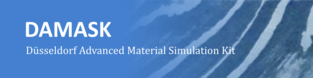 |
DAMASK with grid solvers
Revision: v2.0.3-2204-gdb1f2151
The Düsseldorf Advanced Material Simulation Kit with Grid Solvers
|
|
Go to the documentation of this file. 1 # 1 "/home/damask_user/GitLabCI_Pipeline_4301/DAMASK/src/material.f90"
4 # 1 "/home/damask_user/GitLabCI_Pipeline_4301/DAMASK/src/material.f90"
25 character(len=*),
parameter,
public :: &
54 enum,
bind(c); enumerator :: &
90 integer(kind(ELASTICITY_undefined_ID)),
dimension(:),
allocatable,
public,
protected :: &
92 integer(kind(PLASTICITY_undefined_ID)),
dimension(:),
allocatable,
public,
protected :: &
94 integer(kind(THERMAL_isothermal_ID)),
dimension(:),
allocatable,
public,
protected :: &
96 integer(kind(DAMAGE_none_ID)),
dimension(:),
allocatable,
public,
protected :: &
98 integer(kind(HOMOGENIZATION_undefined_ID)),
dimension(:),
allocatable,
public,
protected :: &
101 integer,
public,
protected :: &
105 integer(kind(SOURCE_undefined_ID)),
dimension(:,:),
allocatable,
public,
protected :: &
106 phase_source, & !< active sources mechanisms of each phase
110 integer,
public,
protected :: &
113 integer,
dimension(:),
allocatable,
public,
protected :: &
114 phase_nsources, & !< number of source mechanisms active in each phase
124 real(
preal),
dimension(:),
allocatable,
public,
protected :: &
128 integer,
dimension(:),
allocatable,
public,
protected :: &
130 integer,
dimension(:,:),
allocatable,
public,
target :: &
132 integer,
dimension(:,:),
allocatable,
public,
protected :: &
134 integer,
dimension(:,:,:),
allocatable,
public,
protected :: &
141 type(
tstate),
allocatable,
dimension(:),
public :: &
146 integer,
dimension(:,:,:),
allocatable,
public,
protected :: &
149 type(
rotation),
dimension(:,:,:),
allocatable,
public,
protected :: &
152 logical,
dimension(:),
allocatable,
public,
protected :: &
155 integer,
dimension(:),
allocatable,
private :: &
158 integer,
dimension(:,:),
allocatable,
private :: &
162 type(
rotation),
dimension(:),
allocatable,
private :: &
218 integer :: i,e,m,c,h, mydebug, myphase, myhomog, mymicro
219 integer,
dimension(:),
allocatable :: &
221 counterhomogenization
225 write(6,
'(/,a)')
' <<<+- material init -+>>>';
flush(6)
228 if (iand(mydebug,
debug_levelbasic) /= 0)
write(6,
'(a)')
' Phase parsed';
flush(6)
231 if (iand(mydebug,
debug_levelbasic) /= 0)
write(6,
'(a)')
' Microstructure parsed';
flush(6)
234 if (iand(mydebug,
debug_levelbasic) /= 0)
write(6,
'(a)')
' Homogenization parsed';
flush(6)
237 if (iand(mydebug,
debug_levelbasic) /= 0)
write(6,
'(a)')
' Texture parsed';
flush(6)
264 call io_error(150,m,ext_msg=
'phase')
267 call io_error(150,m,ext_msg=
'texture')
274 write(6,
'(/,a,/)')
' MATERIAL configuration'
275 write(6,
'(a32,1x,a16,1x,a6)')
'homogenization ',
'type ',
'grains'
279 write(6,
'(/,a14,18x,1x,a11,1x,a12,1x,a13)')
'microstructure',
'constituents'
309 call io_error(150,ext_msg=
'texture')
376 character(len=pStringLen) :: tag
378 logical,
dimension(:),
allocatable :: homogenization_active
398 select case (trim(tag))
409 call io_error(500,ext_msg=trim(tag))
418 select case (trim(tag))
426 call io_error(500,ext_msg=trim(tag))
435 select case (trim(tag))
443 call io_error(500,ext_msg=trim(tag))
466 character(len=pStringLen),
dimension(:),
allocatable :: &
468 integer,
allocatable,
dimension(:) :: chunkPos
470 character(len=pStringLen) :: &
472 real(pReal),
dimension(:,:),
allocatable :: &
473 microstructure_fraction
480 call io_error(155,ext_msg=
'More microstructures in geometry than sections in material.config')
494 do c = 1,
size(strings)
506 microstructure_fraction(c,m) =
io_floatvalue(strings(c),chunkpos,i+1)
523 integer :: sourceCtr, kinematicsCtr, stiffDegradationCtr, p
524 character(len=pStringLen),
dimension(:),
allocatable :: str
574 str = [
'GfortranBug86277']
575 str =
config_phase(p)%getStrings(
'(source)',defaultval=str)
576 if (str(1) ==
'GfortranBug86277') str = [
character(len=pStringLen)::]
580 do sourcectr = 1,
size(str)
581 select case (trim(str(sourcectr)))
598 str = [
'GfortranBug86277']
599 str =
config_phase(p)%getStrings(
'(kinematics)',defaultval=str)
600 if (str(1) ==
'GfortranBug86277') str = [
character(len=pStringLen)::]
604 do kinematicsctr = 1,
size(str)
605 select case (trim(str(kinematicsctr)))
615 str = [
'GfortranBug86277']
616 str =
config_phase(p)%getStrings(
'(stiffness_degradation)',defaultval=str)
617 if (str(1) ==
'GfortranBug86277') str = [
character(len=pStringLen)::]
621 do stiffdegradationctr = 1,
size(str)
622 select case (trim(str(stiffdegradationctr)))
646 character(len=pStringLen),
dimension(:),
allocatable :: strings
647 integer,
dimension(:),
allocatable :: chunkPos
648 real(pReal),
dimension(3,3) :: transformation
649 real(pReal),
dimension(3) :: Eulers
680 select case (strings(j))
682 transformation(j,1:3) = [ 1.0_preal, 0.0_preal, 0.0_preal]
684 transformation(j,1:3) = [-1.0_preal, 0.0_preal, 0.0_preal]
686 transformation(j,1:3) = [ 0.0_preal, 1.0_preal, 0.0_preal]
688 transformation(j,1:3) = [ 0.0_preal,-1.0_preal, 0.0_preal]
690 transformation(j,1:3) = [ 0.0_preal, 0.0_preal, 1.0_preal]
692 transformation(j,1:3) = [ 0.0_preal, 0.0_preal,-1.0_preal]
697 call transformation_%fromMatrix(transformation)
710 sizeState,sizeDotState,sizeDeltaState)
712 integer,
intent(in) :: &
722 plasticstate(phase)%offsetDeltaState = sizestate-sizedeltastate
724 allocate(
plasticstate(phase)%atol (sizestate), source=0.0_preal)
725 allocate(
plasticstate(phase)%state0 (sizestate,nipcmyphase), source=0.0_preal)
726 allocate(
plasticstate(phase)%partionedState0 (sizestate,nipcmyphase), source=0.0_preal)
727 allocate(
plasticstate(phase)%subState0 (sizestate,nipcmyphase), source=0.0_preal)
728 allocate(
plasticstate(phase)%state (sizestate,nipcmyphase), source=0.0_preal)
730 allocate(
plasticstate(phase)%dotState (sizedotstate,nipcmyphase),source=0.0_preal)
732 allocate(
plasticstate(phase)%previousDotState (sizedotstate,nipcmyphase),source=0.0_preal)
733 allocate(
plasticstate(phase)%previousDotState2 (sizedotstate,nipcmyphase),source=0.0_preal)
736 allocate(
plasticstate(phase)%RK4dotState (4,sizedotstate,nipcmyphase),source=0.0_preal)
738 allocate(
plasticstate(phase)%RKCK45dotState (6,sizedotstate,nipcmyphase),source=0.0_preal)
740 allocate(
plasticstate(phase)%deltaState (sizedeltastate,nipcmyphase),source=0.0_preal)
749 sizeState,sizeDotState,sizeDeltaState)
751 integer,
intent(in) :: &
755 sizestate, sizedotstate,sizedeltastate
758 sourcestate(phase)%p(of)%sizeDotState = sizedotstate
759 sourcestate(phase)%p(of)%sizeDeltaState = sizedeltastate
760 sourcestate(phase)%p(of)%offsetDeltaState = sizestate-sizedeltastate
762 allocate(
sourcestate(phase)%p(of)%atol (sizestate), source=0.0_preal)
763 allocate(
sourcestate(phase)%p(of)%state0 (sizestate,nipcmyphase), source=0.0_preal)
764 allocate(
sourcestate(phase)%p(of)%partionedState0 (sizestate,nipcmyphase), source=0.0_preal)
765 allocate(
sourcestate(phase)%p(of)%subState0 (sizestate,nipcmyphase), source=0.0_preal)
766 allocate(
sourcestate(phase)%p(of)%state (sizestate,nipcmyphase), source=0.0_preal)
768 allocate(
sourcestate(phase)%p(of)%dotState (sizedotstate,nipcmyphase),source=0.0_preal)
770 allocate(
sourcestate(phase)%p(of)%previousDotState (sizedotstate,nipcmyphase),source=0.0_preal)
771 allocate(
sourcestate(phase)%p(of)%previousDotState2 (sizedotstate,nipcmyphase),source=0.0_preal)
774 allocate(
sourcestate(phase)%p(of)%RK4dotState (4,sizedotstate,nipcmyphase),source=0.0_preal)
776 allocate(
sourcestate(phase)%p(of)%RKCK45dotState (6,sizedotstate,nipcmyphase),source=0.0_preal)
778 allocate(
sourcestate(phase)%p(of)%deltaState (sizedeltastate,nipcmyphase),source=0.0_preal)
type(thomogmapping), dimension(:), allocatable, public thermalmapping
mapping for thermal state/fields
type(tsourcestate), dimension(:), allocatable, public sourcestate
character(len= *), parameter, public damage_local_label
integer, dimension(:,:), allocatable, public, protected material_phaseat
phase ID of each element
integer, dimension(:), allocatable, public, protected phase_elasticityinstance
instance of particular elasticity of each phase
integer, public, protected numerics_integrator
method used for state integration Default 1: fix-point iteration
rotation storage and conversion
pure integer function, dimension(:), allocatable, public io_stringpos(string)
locates all whitespace-separated chunks in given string and returns array containing number them and ...
@, public source_damage_isoductile_id
type(thomogmapping), dimension(:), allocatable, public damagemapping
mapping for damage state/fields
character(len= *), parameter, public plasticity_isotropic_label
character(len= *), parameter, public plasticity_kinehardening_label
integer, dimension(:,:,:), allocatable, public, protected material_phasememberat
position of the element within its phase instance
subroutine, public results_closejobfile
closes the results file
subroutine, public io_error(error_ID, el, ip, g, instance, ext_msg)
write error statements to standard out and terminate the Marc/spectral run with exit #9xxx
@, public source_damage_isobrittle_id
type(tpartitionedstringlist), dimension(:), allocatable, public, protected config_phase
@, public source_thermal_dissipation_id
@, public kinematics_thermal_expansion_id
integer, dimension(debug_maxntype+2), public, protected debug_level
subroutine, public material_allocateplasticstate(phase, NipcMyPhase, sizeState, sizeDotState, sizeDeltaState)
allocates the plastic state of a phase
character(len= *), parameter, public source_thermal_externalheat_label
integer(kind(plasticity_undefined_id)), dimension(:), allocatable, public, protected phase_plasticity
plasticity of each phase
subroutine, public results_mapping_materialpoint(homogenizationAt, memberAtLocal, label)
adds the unique mapping from spatial position and constituent ID to results
@, public thermal_adiabatic_id
@, public plasticity_isotropic_id
subroutine material_parsetexture
parses the texture part in the material configuration file
@, public damage_nonlocal_id
@, public plasticity_dislotwin_id
type(group_float), dimension(:), allocatable, public temperature
temperature field
character(len=pstringlen), dimension(:), allocatable, public, protected config_name_phase
name of each phase
real(preal), dimension(:), allocatable, public, protected damage_initialphi
initial damage per each homogenization
Parses material config file, either solverJobName.materialConfig or material.config.
integer, public, protected homogenization_maxngrains
max number of grains in any USED homogenization
type(rotation), dimension(:,:,:), allocatable, public, protected material_orientation0
initial orientation of each grain,IP,element
integer, dimension(:,:), allocatable, private microstructure_phase
phase IDs of each microstructure
type(rotation), dimension(:), allocatable, private texture_orientation
Euler angles in material.config (possibly rotated for alignment)
@ plasticity_undefined_id
Reads in the material configuration from file.
integer, dimension(:,:), allocatable, target, private mappinghomogenizationconst
mapping from material points to offset in constant state/field
integer, dimension(:), allocatable, public, protected phase_nkinematics
number of kinematic mechanisms active in each phase
@, public elasticity_hooke_id
type(tstate), dimension(:), allocatable, public damagestate
subroutine, public config_deallocate(what)
deallocates the linked lists that store the content of the configuration files
integer, dimension(:), allocatable, public, protected homogenization_ngrains
number of grains in each homogenization
@, public thermal_isothermal_id
real(preal), dimension(:), allocatable, public, protected thermal_initialt
initial temperature per each homogenization
@, public damage_local_id
character(len= *), parameter, public thermal_conduction_label
integer(kind(elasticity_undefined_id)), dimension(:), allocatable, public, protected phase_elasticity
elasticity of each phase
@ kinematics_undefined_id
integer(kind(thermal_isothermal_id)), dimension(:), allocatable, public, protected thermal_type
thermal transport model
setting precision for real and int type
integer, dimension(:), allocatable, public, protected homogenization_typeinstance
instance of particular type of each homogenization
integer, parameter, public debug_material
stores debug level for material part of DAMASK bitwise coded
type(tstate), dimension(:), allocatable, public homogstate
character(len=pstringlen), dimension(:), allocatable, public, protected config_name_microstructure
name of each microstructure
@ homogenization_undefined_id
integer, dimension(:), allocatable, public, protected discretization_homogenizationat
character(len= *), parameter, public source_damage_isobrittle_label
character(len= *), parameter, public homogenization_rgc_label
integer, dimension(:,:), allocatable, private microstructure_texture
texture IDs of each microstructure
subroutine material_parsemicrostructure
parses the microstructure part in the material configuration file
integer, public, protected discretization_nip
character(len= *), parameter, public homogenization_none_label
@, public source_thermal_externalheat_id
@, public homogenization_isostrain_id
character(len= *), parameter, public kinematics_cleavage_opening_label
character(len= *), parameter, public thermal_isothermal_label
@, public plasticity_phenopowerlaw_id
logical, dimension(:), allocatable, public, protected phase_localplasticity
flags phases with local constitutive law
input/output functions, partly depending on chosen solver
integer, dimension(:), allocatable, public, protected damage_typeinstance
instance of particular type of each nonlocal damage
character(len=pstringlen), dimension(:), allocatable, public, protected config_name_texture
name of each texture
integer, dimension(:), allocatable, public, protected phase_plasticityinstance
instance of particular plasticity of each phase
character(len= *), parameter, public plasticity_none_label
integer, parameter, public debug_levelbasic
integer, parameter preal
number with 15 significant digits, up to 1e+-307 (typically 64 bit)
Reading in and interpretating the debugging settings for the various modules.
@, public kinematics_slipplane_opening_id
subroutine, public material_allocatesourcestate(phase, of, NipcMyPhase, sizeState, sizeDotState, sizeDeltaState)
allocates the source state of a phase
integer(kind(source_undefined_id)), dimension(:,:), allocatable, public, protected phase_kinematics
active kinematic mechanisms of each phase
type(tpartitionedstringlist), dimension(:), allocatable, public, protected config_texture
@, public source_damage_anisobrittle_id
integer, dimension(:), allocatable, public, protected phase_nstiffnessdegradations
number of stiffness degradation mechanisms active in each phase
character(len=pstringlen), dimension(:), allocatable, public, protected config_name_homogenization
name of each homogenization
subroutine, public results_mapping_constituent(phaseAt, memberAtLocal, label)
adds the unique mapping from spatial position and constituent ID to results
type(tstate), dimension(:), allocatable, public thermalstate
character(len= *), parameter, public elasticity_hooke_label
logical elemental pure function dneq(a, b, tol)
inequality comparison for float with double precision
character(len= *), parameter, public source_damage_anisobrittle_label
integer, parameter, public debug_levelextensive
variable length datatype used for storage of state
character(len= *), parameter, public damage_nonlocal_label
integer, dimension(:), allocatable, public, protected thermal_typeinstance
instance of particular type of each thermal transport
@ elasticity_undefined_id
integer function, public io_intvalue(string, chunkPos, myChunk)
reads integer value at myChunk from string
character(len= *), parameter, public stiffness_degradation_damage_label
subroutine, public results_openjobfile
opens the results file to append data
@, public stiffness_degradation_damage_id
@, public plasticity_disloucla_id
integer, dimension(:), allocatable, public, protected material_homogenizationat
homogenization ID of each element (copy of discretization_homogenizationAt)
integer, public, protected material_nphase
number of phases
subroutine, public material_init
parses material configuration file
character(len= *), parameter, public kinematics_slipplane_opening_label
type(tplasticstate), dimension(:), allocatable, public plasticstate
Mathematical library, including random number generation and tensor representations.
character(len= *), parameter, public source_damage_anisoductile_label
character(len= *), parameter, public plasticity_dislotwin_label
@ stiffness_degradation_undefined_id
type(tpartitionedstringlist), dimension(:), allocatable, public, protected config_microstructure
integer, public, protected material_nhomogenization
number of homogenizations
@, public kinematics_cleavage_opening_id
character(len= *), parameter, public plasticity_nonlocal_label
character(len= *), parameter, public kinematics_thermal_expansion_label
@, public plasticity_nonlocal_id
integer, public, protected discretization_nelem
@, public plasticity_kinehardening_id
character(len= *), parameter, public damage_none_label
integer, dimension(:), allocatable, public, protected discretization_microstructureat
integer, dimension(:,:,:), allocatable, public, protected material_texture
texture (index) of each grain,IP,element. Only used by plastic_nonlocal
integer(kind(source_undefined_id)), dimension(:,:), allocatable, public, protected phase_source
active sources mechanisms of each phase
integer, dimension(:,:), allocatable, target, public material_homogenizationmemberat
position of the element within its homogenization instance
subroutine material_parsehomogenization
parses the homogenization part from the material configuration
integer(kind(homogenization_undefined_id)), dimension(:), allocatable, public, protected homogenization_type
type of each homogenization
integer(kind(source_undefined_id)), dimension(:,:), allocatable, public, protected phase_stiffnessdegradation
active stiffness degradation mechanisms of each phase
character(len=:) function, allocatable, public io_stringvalue(string, chunkPos, myChunk)
reads string value at myChunk from string
integer, dimension(:), allocatable, public, protected phase_nsources
number of source mechanisms active in each phase
character(len= *), parameter, public plasticity_disloucla_label
character(len= *), parameter, public plasticity_phenopowerlaw_label
type(group_float), dimension(:), allocatable, public damage
damage field
character(len= *), parameter, public thermal_adiabatic_label
integer(kind(damage_none_id)), dimension(:), allocatable, public, protected damage_type
nonlocal damage model
Managing of parameters related to numerics.
type(group_float), dimension(:), allocatable, public temperaturerate
temperature change rate field
integer, dimension(:), allocatable, private microstructure_nconstituents
number of constituents in each microstructure
@, public source_damage_anisoductile_id
@, public plasticity_none_id
character(len= *), parameter, public source_damage_isoductile_label
type(tpartitionedstringlist), dimension(:), allocatable, public, protected config_homogenization
@, public homogenization_rgc_id
@, public homogenization_none_id
real(preal) function, public io_floatvalue(string, chunkPos, myChunk)
reads float value at myChunk from string
@, public thermal_conduction_id
subroutine material_parsephase
parses the phase part in the material configuration file
character(len= *), parameter, public homogenization_isostrain_label
character(len= *), parameter, public source_thermal_dissipation_label