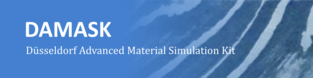 |
DAMASK with grid solvers
Revision: v2.0.3-2204-gdb1f2151
The Düsseldorf Advanced Material Simulation Kit with Grid Solvers
|
|
Go to the documentation of this file. 1 # 1 "/home/damask_user/GitLabCI_Pipeline_4301/DAMASK/src/kinematics_thermal_expansion.f90"
4 # 1 "/home/damask_user/GitLabCI_Pipeline_4301/DAMASK/src/kinematics_thermal_expansion.f90"
27 real(
preal),
dimension(3,3,3) :: &
47 integer :: ninstance,p,i
48 real(
preal),
dimension(:),
allocatable :: temp
54 write(6,
'(a16,1x,i5,/)')
'# instances:',ninstance
57 allocate(
param(ninstance))
66 prm%T_ref =
config%getFloat(
'reference_temperature', defaultval=0.0_preal)
69 temp =
config%getFloats(
'thermal_expansion11')
70 prm%expansion(1,1,1:
size(temp)) = temp
71 temp =
config%getFloats(
'thermal_expansion22',defaultval=[(0.0_preal, i=1,
size(temp))],requiredsize=
size(temp))
72 prm%expansion(2,2,1:
size(temp)) = temp
73 temp =
config%getFloats(
'thermal_expansion33',defaultval=[(0.0_preal, i=1,
size(temp))],requiredsize=
size(temp))
74 prm%expansion(3,3,1:
size(temp)) = temp
75 do i=1,
size(prm%expansion,3)
90 integer,
intent(in) :: &
95 real(preal),
dimension(3,3) :: &
100 (temperature(homog)%p(offset) - prm%T_ref)**1 / 1. * prm%expansion(1:3,1:3,1) + &
101 (temperature(homog)%p(offset) - prm%T_ref)**2 / 2. * prm%expansion(1:3,1:3,2) + &
102 (temperature(homog)%p(offset) - prm%T_ref)**3 / 3. * prm%expansion(1:3,1:3,3)
113 integer,
intent(in) :: &
114 ipc, & !< grain number
115 ip, & !< integration point number
117 real(preal),
intent(out),
dimension(3,3) :: &
119 real(preal),
intent(out),
dimension(3,3,3,3) :: &
128 phase = material_phaseat(ipc,el)
129 homog = material_homogenizationat(el)
130 t = temperature(homog)%p(thermalmapping(homog)%p(ip,el))
131 tdot = temperaturerate(homog)%p(thermalmapping(homog)%p(ip,el))
135 prm%expansion(1:3,1:3,1)*(t - prm%T_ref)**0 &
136 + prm%expansion(1:3,1:3,2)*(t - prm%T_ref)**1 &
137 + prm%expansion(1:3,1:3,3)*(t - prm%T_ref)**2 &
140 + prm%expansion(1:3,1:3,1)*(t - prm%T_ref)**1 / 1. &
141 + prm%expansion(1:3,1:3,2)*(t - prm%T_ref)**2 / 2. &
142 + prm%expansion(1:3,1:3,3)*(t - prm%T_ref)**3 / 3. &
145 dli_dtstar = 0.0_preal
subroutine, public kinematics_thermal_expansion_lianditstangent(Li, dLi_dTstar, ipc, ip, el)
contains the constitutive equation for calculating the velocity gradient
pure real(preal) function, dimension(3, 3), public kinematics_thermal_expansion_initialstrain(homog, phase, offset)
report initial thermal strain based on current temperature deviation from reference
type(tpartitionedstringlist), dimension(:), allocatable, public, protected config_phase
@, public kinematics_thermal_expansion_id
integer, dimension(debug_maxntype+2), public, protected debug_level
subroutine, public kinematics_thermal_expansion_init
module initialization
Parses material config file, either solverJobName.materialConfig or material.config.
Reads in the material configuration from file.
real(preal) function, dimension(3, 3), public lattice_applylatticesymmetry33(T, structure)
Return 3x3 tensor with symmetry according to given crystal structure.
material subroutine incorporating kinematics resulting from thermal expansion
setting precision for real and int type
input/output functions, partly depending on chosen solver
integer, parameter, public debug_levelbasic
integer, parameter preal
number with 15 significant digits, up to 1e+-307 (typically 64 bit)
Reading in and interpretating the debugging settings for the various modules.
integer(kind(source_undefined_id)), dimension(:,:), allocatable, public, protected phase_kinematics
active kinematic mechanisms of each phase
contains lattice structure definitions including Schmid matrices for slip, twin, trans,
Mathematical library, including random number generation and tensor representations.
integer, parameter, public debug_constitutive
stores debug level for constitutive part of DAMASK bitwise coded
character(len= *), parameter, public kinematics_thermal_expansion_label
integer, dimension(:), allocatable kinematics_thermal_expansion_instance
type(tparameters), dimension(:), allocatable param